java.lang.Object
javafx.scene.Node
javafx.scene.Parent
javafx.scene.layout.Region
javafx.scene.control.Control
com.flexganttfx.view.util.FlexGanttFXControl
com.flexganttfx.view.GanttChartBase<R>
com.flexganttfx.view.GanttChart<R>
- Type Parameters:
R
- the type of the rows shown by the Gantt chart (e.g. "Aircraft")
- All Implemented Interfaces:
Styleable
,EventTarget
,Skinnable
public class GanttChart<R extends Row<?,?,?>> extends GanttChartBase<R>
A control used to visualize any kind of scheduling data along a
timeline. The model data needed by the control consists of rows with
activities, links between activities, and layers to group activities
together.
setRoot(Row)
- sets the root row.GanttChartBase.getLayers()
- returns the list of layersGanttChartBase.getLinks()
- returns the list of links between activities
The control consists of several children controls:
TreeTableView
: shown on the left-hand side to display a hierarchical structure of rowsGraphicsBase
: shown on the right-hand side to display a graphical representation of the model dataTimeline
: shown above the graphics view. The timeline itself consists of two child controls.Dateline
: displays days, weeks, months, years, etc...Eventline
: displays various time markers
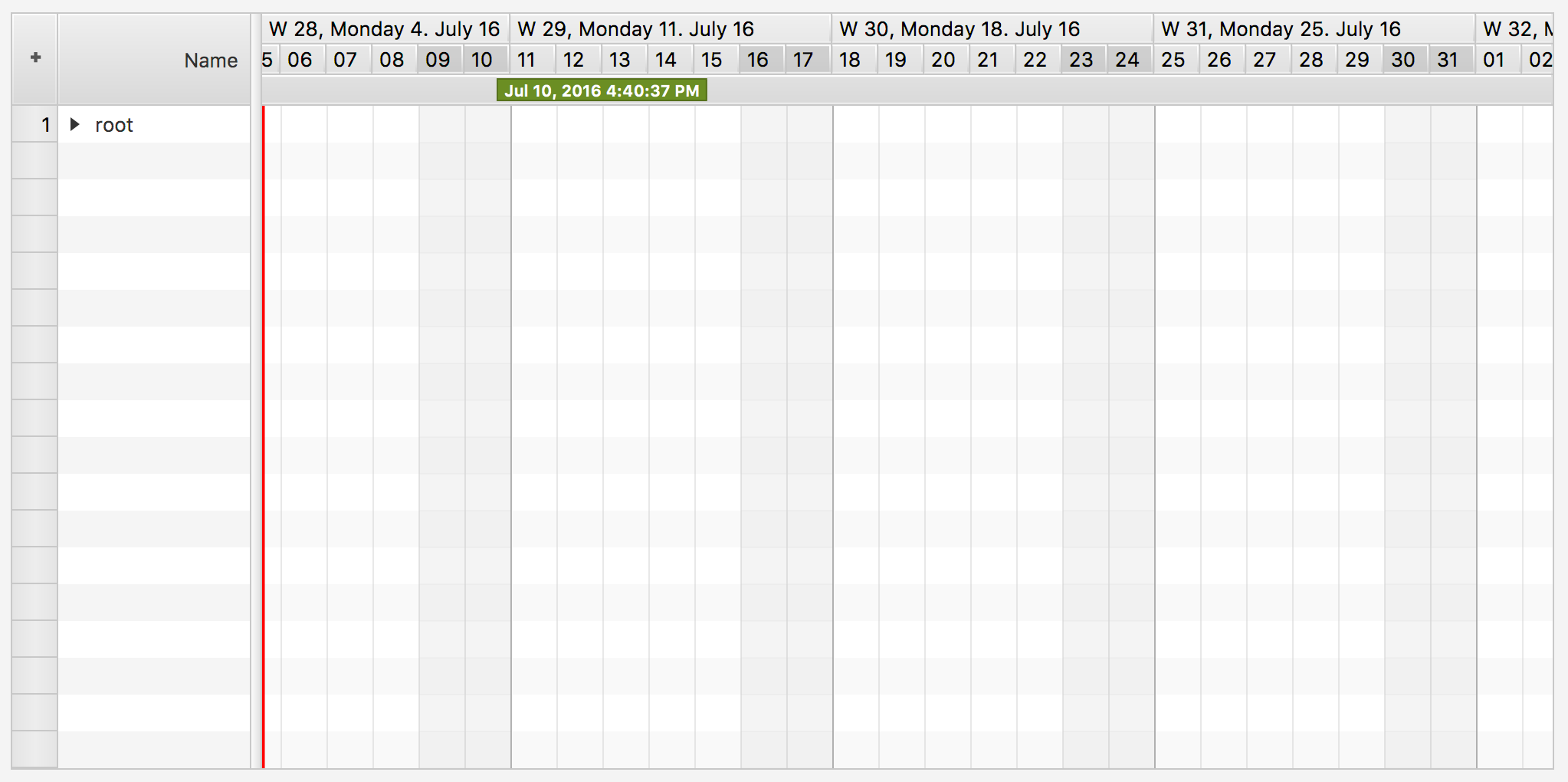
Master / Detail Panes
The Gantt chart uses two MasterDetailPane instances from ControlsFX for the high-level layout. The tree table master detail pane displays the tree table as its detail node. The graphics master detail pane displays a property sheet as its detail node. The property sheet is used at development time and can be replaced with any node by callingGanttChartBase.setDetail(Node)
. The property
sheet displays a lot of properties that are used by the controls, the
renderers, the system layers to fine-tune the appearance of the control. Many
of them can be changed at runtime.
Standalone vs. Multi- / Dual Gantt Chart
A Gantt chart can be used standalone or inside aMultiGanttChartContainer
or
DualGanttChartContainer
. When used in one of these containers the
Position
of the Gantt chart becomes important. The control can be the
first chart, the last chart, the only chart, or a chart somewhere in the
middle. A "first" or "only" chart always displays a timeline. A "middle" or
"last" displays a special header (see GanttChartBase.setGraphicsHeader(Node)
). The
containers are also the reason why the control distinguishes between a
timeline (GanttChartBase.getTimeline()
) and a master timeline (
GanttChartBase.getMasterTimeline()
). The master timeline is the one shown by the
"first" chart, while the regular timeline is the one that belongs directly to
an individual Gantt chart instance.
Code Example
import java.time.Duration; import java.time.Instant; import java.time.temporal.ChronoUnit; import javafx.application.Application; import javafx.scene.Scene; import javafx.stage.Stage; import com.flexganttfx.model.GanttChartModel; import com.flexganttfx.model.Layer; import com.flexganttfx.model.Row; import com.flexganttfx.model.activity.MutableActivityBase; import com.flexganttfx.model.layout.GanttLayout; import com.flexganttfx.view.GanttChart; import com.flexganttfx.view.graphics.GraphicsView; import com.flexganttfx.view.graphics.renderer.ActivityBarRenderer; import com.flexganttfx.view.timeline.Timeline; public class TutorialAircraftFlight extends Application { class FlightData { String flightNo; Instant departureTime = Instant.now(); Instant arrivalTime = Instant.now().plus(Duration.ofHours(6)); public FlightData(String flightNo, int day) { this.flightNo = flightNo; departureTime = departureTime.plus(Duration.ofDays(day)); arrivalTime = arrivalTime.plus(Duration.ofDays(day)); } } class Flight extends MutableActivityBase<FlightData> { public Flight(FlightData data) { setUserObject(data); setName(data.flightNo); setStartTime(data.departureTime); setEndTime(data.arrivalTime); } } class Aircraft extends Row<Aircraft, Aircraft, Flight> { public Aircraft(String name) { super(name); } } public void start(Stage stage) { // Create the root row Aircraft root = new Aircraft("Root"); root.setExpanded(true); // Create the Gantt chart GanttChart<Aircraft> gantt = new GanttChart<>(new FlightSchedule(new Aircraft("ROOT"))); Layer flightsLayer = new Layer("Flights"); gantt.getLayers().add(flightsLayer); Aircraft b747 = new Aircraft("B747"); b747.addActivity(flightsLayer, new Flight(new FlightData("flight1", 1))); b747.addActivity(flightsLayer, new Flight(new FlightData("flight2", 2))); b747.addActivity(flightsLayer, new Flight(new FlightData("flight3", 3))); Aircraft a380 = new Aircraft("A380"); a380.addActivity(flightsLayer, new Flight(new FlightData("flight1", 1))); a380.addActivity(flightsLayer, new Flight(new FlightData("flight2", 2))); a380.addActivity(flightsLayer, new Flight(new FlightData("flight3", 3))); root.getChildren().setAll(b747, a380); Timeline timeline = gantt.getTimeline(); timeline.showTemporalUnit(ChronoUnit.HOURS, 10); GraphicsView<Aircraft> graphics = gantt.getGraphics(); graphics.setActivityRenderer(Flight.class, GanttLayout.class, new ActivityBarRenderer<>(graphics, "Flight Renderer")); graphics.showEarliestActivities(); Scene scene = new Scene(gantt); stage.setScene(scene); stage.sizeToScene(); stage.centerOnScreen(); stage.show(); } public static void main(String[] args) { launch(args); }
- Since:
- 1.0
-
Property Summary
Properties Type Property Description ObjectProperty<GanttChart.DisplayMode>
displayMode
A property used to specify the mode in which the Gantt chart will layout its primary views, the table and the graphics.ObjectProperty<R>
root
Returns the root row property.ObjectProperty<Callback<R,Node>>
rowHeaderNodeFactory
A property used to store a callback for creating a node that will be placed to the left of each row in the tree table view.ObjectProperty<GanttChart.RowHeaderType>
rowHeaderType
A property used to store the currently used type of row headers (row number, level number, custom graphics).BooleanProperty
showTreeTable
A property used to control whether the tree table view will be shown or not.BooleanProperty
tableMenuButtonVisible
This controls whether a menu button is available when the user clicks in a designated space within the TreeTableView, within which is a check menu item for each column in this table.Properties inherited from class com.flexganttfx.view.GanttChartBase
autoHideScrollBar, detail, fixedCellSize, graphicsHeader, masterTimeline, position, rowFilter, scrollBarType, showDetail
Properties inherited from class javafx.scene.control.Control
contextMenu, skin, tooltip
Properties inherited from class javafx.scene.layout.Region
background, border, cacheShape, centerShape, height, insets, maxHeight, maxWidth, minHeight, minWidth, opaqueInsets, padding, prefHeight, prefWidth, scaleShape, shape, snapToPixel, width
Properties inherited from class javafx.scene.Parent
needsLayout
Properties inherited from class javafx.scene.Node
accessibleHelp, accessibleRoleDescription, accessibleRole, accessibleText, blendMode, boundsInLocal, boundsInParent, cacheHint, cache, clip, cursor, depthTest, disabled, disable, effectiveNodeOrientation, effect, eventDispatcher, focused, focusTraversable, hover, id, inputMethodRequests, layoutBounds, layoutX, layoutY, localToParentTransform, localToSceneTransform, managed, mouseTransparent, nodeOrientation, onContextMenuRequested, onDragDetected, onDragDone, onDragDropped, onDragEntered, onDragExited, onDragOver, onInputMethodTextChanged, onKeyPressed, onKeyReleased, onKeyTyped, onMouseClicked, onMouseDragEntered, onMouseDragExited, onMouseDragged, onMouseDragOver, onMouseDragReleased, onMouseEntered, onMouseExited, onMouseMoved, onMousePressed, onMouseReleased, onRotate, onRotationFinished, onRotationStarted, onScrollFinished, onScroll, onScrollStarted, onSwipeDown, onSwipeLeft, onSwipeRight, onSwipeUp, onTouchMoved, onTouchPressed, onTouchReleased, onTouchStationary, onZoomFinished, onZoom, onZoomStarted, opacity, parent, pickOnBounds, pressed, rotate, rotationAxis, scaleX, scaleY, scaleZ, scene, style, translateX, translateY, translateZ, viewOrder, visible
-
Nested Class Summary
Nested Classes Modifier and Type Class Description static class
GanttChart.DisplayMode
An enum used for specifying how to layout the Gantt chart.static class
GanttChart.RowHeaderType
An enum used to control the visuals of the cells in the row header column.Nested classes/interfaces inherited from class com.flexganttfx.view.GanttChartBase
GanttChartBase.ScrollBarType
-
Field Summary
Fields inherited from class javafx.scene.layout.Region
USE_COMPUTED_SIZE, USE_PREF_SIZE
Fields inherited from class javafx.scene.Node
BASELINE_OFFSET_SAME_AS_HEIGHT
-
Constructor Summary
Constructors Constructor Description GanttChart()
Constructs a new Gantt chart control.GanttChart(R root)
Constructs a new Gantt Chart control. -
Method Summary
Modifier and Type Method Description void
collapseRows()
Collapses all rows inside the Gantt chart.void
collapseRowsByOneLevel()
Collapses the hightest level of rows inside the Gantt chart that is currently being used.protected Skin<?>
createDefaultSkin()
protected RowHeaderColumn<R>
createRowHeaderColumn()
Creates the row header column used by the Gantt chart.protected TreeTableView<R>
createTreeTable()
Creates the tree table view instance.ObjectProperty<GanttChart.DisplayMode>
displayModeProperty()
A property used to specify the mode in which the Gantt chart will layout its primary views, the table and the graphics.void
expandRows()
Expands all rows inside the Gantt chart.void
expandRowsByOneLevel()
Expands the next level of rows inside the Gantt chart.GanttChart.DisplayMode
getDisplayMode()
Returns the value ofdisplayModeProperty()
.R
getRoot()
Returns the root row of the Gantt chart.RowHeaderColumn<R>
getRowHeaderColumn()
Returns the row header control used as the first column of the tree table view.Callback<R,Node>
getRowHeaderNodeFactory()
Returns the value ofrowHeaderNodeFactoryProperty()
.GanttChart.RowHeaderType
getRowHeaderType()
Returns the value ofrowHeaderTypeProperty()
.TreeTableView<R>
getTreeTable()
Returns theTreeTableView
instance that is shown on the left-hand side of the Gantt chart.org.controlsfx.control.MasterDetailPane
getTreeTableMasterDetailPane()
Returns the primaryMasterDetailPane
instance that is being used to display theTreeTableView
and theListViewGraphics
.ScrollBar
getTreeTableScrollBar()
Returns the scrollbar that is being used for horizontal scrolling operations of the tree table view.boolean
isShowTreeTable()
Returns the value ofshowTreeTableProperty()
.boolean
isTableMenuButtonVisible()
Returns the value oftableMenuButtonVisibleProperty()
.void
resizeColumn(TreeTableColumn<R,?> column)
This method will resize the given column in the tree table view to ensure that the content of the column cells will be completely visible.void
resizeColumn(TreeTableColumn tc, int maxRows)
This method will resize the given column in the tree table view to ensure that the content of the column cells will be completely visible.void
resizeColumns()
This method will resize all columns in the tree table view to ensure that the content of all cells will be completely visible.void
resizeColumns(int maxRows)
This method will resize all columns in the tree table view to ensure that the content of all cells will be completely visible.ObjectProperty<R>
rootProperty()
Returns the root row property.ObjectProperty<Callback<R,Node>>
rowHeaderNodeFactoryProperty()
A property used to store a callback for creating a node that will be placed to the left of each row in the tree table view.ObjectProperty<GanttChart.RowHeaderType>
rowHeaderTypeProperty()
A property used to store the currently used type of row headers (row number, level number, custom graphics).void
setDisplayMode(GanttChart.DisplayMode mode)
Sets the value of thedisplayModeProperty()
.void
setRoot(R root)
Sets a new root on the Gantt chart, which will cause the framework to set a new root of typeGanttChartTreeItem
on the underlyingTreeTableView
.void
setRowHeaderNodeFactory(Callback<R,Node> factory)
Sets the value ofrowHeaderNodeFactoryProperty()
.void
setRowHeaderType(GanttChart.RowHeaderType type)
Sets the value ofrowHeaderTypeProperty()
.void
setShowTreeTable(boolean show)
Sets the value ofshowTreeTableProperty()
.void
setTableMenuButtonVisible(boolean value)
Sets the value oftableMenuButtonVisibleProperty()
.BooleanProperty
showTreeTableProperty()
A property used to control whether the tree table view will be shown or not.BooleanProperty
tableMenuButtonVisibleProperty()
This controls whether a menu button is available when the user clicks in a designated space within the TreeTableView, within which is a check menu item for each column in this table.Methods inherited from class com.flexganttfx.view.GanttChartBase
autoHideScrollBarProperty, createGraphics, createHorizonScrollBar, createTimeline, createTimelineScrollBar, detailProperty, fixedCellSizeProperty, getCalendars, getDetail, getFixedCellSize, getGraphics, getGraphicsHeader, getGraphicsMasterDetailPane, getHorizonScrollBar, getLayers, getLinks, getMasterTimeline, getPosition, getRowFilter, getScrollBarType, getTimeline, getTimelineScrollBar, getUserAgentStylesheet, graphicsHeaderProperty, isAutoHideScrollBar, isShowDetail, masterTimelineProperty, positionProperty, redrawObservable, rowFilterProperty, scrollBarTypeProperty, setAutoHideScrollBar, setDetail, setFixedCellSize, setGraphicsHeader, setMasterTimeline, setPosition, setRowFilter, setScrollBarType, setShowDetail, showDetailProperty
Methods inherited from class com.flexganttfx.view.util.FlexGanttFXControl
getUserAgentStylesheet
Methods inherited from class javafx.scene.control.Control
computeMaxHeight, computeMaxWidth, computeMinHeight, computeMinWidth, computePrefHeight, computePrefWidth, contextMenuProperty, executeAccessibleAction, getBaselineOffset, getClassCssMetaData, getContextMenu, getControlCssMetaData, getCssMetaData, getInitialFocusTraversable, getSkin, getTooltip, isResizable, layoutChildren, queryAccessibleAttribute, setContextMenu, setSkin, setTooltip, skinProperty, tooltipProperty
Methods inherited from class javafx.scene.layout.Region
backgroundProperty, borderProperty, cacheShapeProperty, centerShapeProperty, getBackground, getBorder, getHeight, getInsets, getMaxHeight, getMaxWidth, getMinHeight, getMinWidth, getOpaqueInsets, getPadding, getPrefHeight, getPrefWidth, getShape, getWidth, heightProperty, insetsProperty, isCacheShape, isCenterShape, isScaleShape, isSnapToPixel, layoutInArea, layoutInArea, layoutInArea, layoutInArea, maxHeight, maxHeightProperty, maxWidth, maxWidthProperty, minHeight, minHeightProperty, minWidth, minWidthProperty, opaqueInsetsProperty, paddingProperty, positionInArea, positionInArea, prefHeight, prefHeightProperty, prefWidth, prefWidthProperty, resize, scaleShapeProperty, setBackground, setBorder, setCacheShape, setCenterShape, setHeight, setMaxHeight, setMaxSize, setMaxWidth, setMinHeight, setMinSize, setMinWidth, setOpaqueInsets, setPadding, setPrefHeight, setPrefSize, setPrefWidth, setScaleShape, setShape, setSnapToPixel, setWidth, shapeProperty, snappedBottomInset, snappedLeftInset, snappedRightInset, snappedTopInset, snapPosition, snapPositionX, snapPositionY, snapSize, snapSizeX, snapSizeY, snapSpace, snapSpaceX, snapSpaceY, snapToPixelProperty, widthProperty
Methods inherited from class javafx.scene.Parent
getChildren, getChildrenUnmodifiable, getManagedChildren, getStylesheets, isNeedsLayout, layout, lookup, needsLayoutProperty, requestLayout, requestParentLayout, setNeedsLayout, updateBounds
Methods inherited from class javafx.scene.Node
accessibleHelpProperty, accessibleRoleDescriptionProperty, accessibleRoleProperty, accessibleTextProperty, addEventFilter, addEventHandler, applyCss, autosize, blendModeProperty, boundsInLocalProperty, boundsInParentProperty, buildEventDispatchChain, cacheHintProperty, cacheProperty, clipProperty, computeAreaInScreen, contains, contains, cursorProperty, depthTestProperty, disabledProperty, disableProperty, effectiveNodeOrientationProperty, effectProperty, eventDispatcherProperty, fireEvent, focusedProperty, focusTraversableProperty, getAccessibleHelp, getAccessibleRole, getAccessibleRoleDescription, getAccessibleText, getBlendMode, getBoundsInLocal, getBoundsInParent, getCacheHint, getClip, getContentBias, getCursor, getDepthTest, getEffect, getEffectiveNodeOrientation, getEventDispatcher, getId, getInitialCursor, getInputMethodRequests, getLayoutBounds, getLayoutX, getLayoutY, getLocalToParentTransform, getLocalToSceneTransform, getNodeOrientation, getOnContextMenuRequested, getOnDragDetected, getOnDragDone, getOnDragDropped, getOnDragEntered, getOnDragExited, getOnDragOver, getOnInputMethodTextChanged, getOnKeyPressed, getOnKeyReleased, getOnKeyTyped, getOnMouseClicked, getOnMouseDragEntered, getOnMouseDragExited, getOnMouseDragged, getOnMouseDragOver, getOnMouseDragReleased, getOnMouseEntered, getOnMouseExited, getOnMouseMoved, getOnMousePressed, getOnMouseReleased, getOnRotate, getOnRotationFinished, getOnRotationStarted, getOnScroll, getOnScrollFinished, getOnScrollStarted, getOnSwipeDown, getOnSwipeLeft, getOnSwipeRight, getOnSwipeUp, getOnTouchMoved, getOnTouchPressed, getOnTouchReleased, getOnTouchStationary, getOnZoom, getOnZoomFinished, getOnZoomStarted, getOpacity, getParent, getProperties, getPseudoClassStates, getRotate, getRotationAxis, getScaleX, getScaleY, getScaleZ, getScene, getStyle, getStyleableParent, getStyleClass, getTransforms, getTranslateX, getTranslateY, getTranslateZ, getTypeSelector, getUserData, getViewOrder, hasProperties, hoverProperty, idProperty, inputMethodRequestsProperty, intersects, intersects, isCache, isDisable, isDisabled, isFocused, isFocusTraversable, isHover, isManaged, isMouseTransparent, isPickOnBounds, isPressed, isVisible, layoutBoundsProperty, layoutXProperty, layoutYProperty, localToParent, localToParent, localToParent, localToParent, localToParent, localToParentTransformProperty, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToScene, localToSceneTransformProperty, localToScreen, localToScreen, localToScreen, localToScreen, localToScreen, lookupAll, managedProperty, mouseTransparentProperty, nodeOrientationProperty, notifyAccessibleAttributeChanged, onContextMenuRequestedProperty, onDragDetectedProperty, onDragDoneProperty, onDragDroppedProperty, onDragEnteredProperty, onDragExitedProperty, onDragOverProperty, onInputMethodTextChangedProperty, onKeyPressedProperty, onKeyReleasedProperty, onKeyTypedProperty, onMouseClickedProperty, onMouseDragEnteredProperty, onMouseDragExitedProperty, onMouseDraggedProperty, onMouseDragOverProperty, onMouseDragReleasedProperty, onMouseEnteredProperty, onMouseExitedProperty, onMouseMovedProperty, onMousePressedProperty, onMouseReleasedProperty, onRotateProperty, onRotationFinishedProperty, onRotationStartedProperty, onScrollFinishedProperty, onScrollProperty, onScrollStartedProperty, onSwipeDownProperty, onSwipeLeftProperty, onSwipeRightProperty, onSwipeUpProperty, onTouchMovedProperty, onTouchPressedProperty, onTouchReleasedProperty, onTouchStationaryProperty, onZoomFinishedProperty, onZoomProperty, onZoomStartedProperty, opacityProperty, parentProperty, parentToLocal, parentToLocal, parentToLocal, parentToLocal, parentToLocal, pickOnBoundsProperty, pressedProperty, pseudoClassStateChanged, relocate, removeEventFilter, removeEventHandler, requestFocus, resizeRelocate, rotateProperty, rotationAxisProperty, scaleXProperty, scaleYProperty, scaleZProperty, sceneProperty, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, sceneToLocal, screenToLocal, screenToLocal, screenToLocal, setAccessibleHelp, setAccessibleRole, setAccessibleRoleDescription, setAccessibleText, setBlendMode, setCache, setCacheHint, setClip, setCursor, setDepthTest, setDisable, setDisabled, setEffect, setEventDispatcher, setEventHandler, setFocused, setFocusTraversable, setHover, setId, setInputMethodRequests, setLayoutX, setLayoutY, setManaged, setMouseTransparent, setNodeOrientation, setOnContextMenuRequested, setOnDragDetected, setOnDragDone, setOnDragDropped, setOnDragEntered, setOnDragExited, setOnDragOver, setOnInputMethodTextChanged, setOnKeyPressed, setOnKeyReleased, setOnKeyTyped, setOnMouseClicked, setOnMouseDragEntered, setOnMouseDragExited, setOnMouseDragged, setOnMouseDragOver, setOnMouseDragReleased, setOnMouseEntered, setOnMouseExited, setOnMouseMoved, setOnMousePressed, setOnMouseReleased, setOnRotate, setOnRotationFinished, setOnRotationStarted, setOnScroll, setOnScrollFinished, setOnScrollStarted, setOnSwipeDown, setOnSwipeLeft, setOnSwipeRight, setOnSwipeUp, setOnTouchMoved, setOnTouchPressed, setOnTouchReleased, setOnTouchStationary, setOnZoom, setOnZoomFinished, setOnZoomStarted, setOpacity, setPickOnBounds, setPressed, setRotate, setRotationAxis, setScaleX, setScaleY, setScaleZ, setStyle, setTranslateX, setTranslateY, setTranslateZ, setUserData, setViewOrder, setVisible, snapshot, snapshot, startDragAndDrop, startFullDrag, styleProperty, toBack, toFront, toString, translateXProperty, translateYProperty, translateZProperty, usesMirroring, viewOrderProperty, visibleProperty
Methods inherited from class java.lang.Object
clone, equals, finalize, getClass, hashCode, notify, notifyAll, wait, wait, wait
Methods inherited from interface javafx.css.Styleable
getStyleableNode
-
Property Details
-
displayMode
A property used to specify the mode in which the Gantt chart will layout its primary views, the table and the graphics. Using this property the application can quickly switch between a standard, table-only, or graphics-only display.- Since:
- 1.0
- See Also:
getDisplayMode()
,setDisplayMode(GanttChart.DisplayMode)
-
tableMenuButtonVisible
This controls whether a menu button is available when the user clicks in a designated space within the TreeTableView, within which is a check menu item for each column in this table. This menu allows for the user to show and hide all TreeTableColumns easily.- Since:
- 1.0
- See Also:
isTableMenuButtonVisible()
,setTableMenuButtonVisible(boolean)
-
root
Returns the root row property. The root row will become the root node of the Gantt chart's tree table control on the left-hand side (wrapped inside an instance of typeGanttChartTreeItem
). Other rows can be added by adding them to the root row or one of its children.- Since:
- 1.0
- See Also:
getRoot()
,setRoot(R)
-
showTreeTable
A property used to control whether the tree table view will be shown or not. This node gets shown on the left-hand side of the Gantt chart and dispays a hierarchy (e.g. a resource hierarchy). The tree table view is the detail node of the primary master detail pane (seegetTreeTableMasterDetailPane()
).- Since:
- 1.0
- See Also:
isShowTreeTable()
,setShowTreeTable(boolean)
-
rowHeaderType
A property used to store the currently used type of row headers (row number, level number, custom graphics).- Since:
- 1.0
- See Also:
getRowHeaderType()
,setRowHeaderType(GanttChart.RowHeaderType)
-
rowHeaderNodeFactory
A property used to store a callback for creating a node that will be placed to the left of each row in the tree table view. This factory will only be invoked if the row header type is set toGanttChart.RowHeaderType.GRAPHIC_NODE
.- Since:
- 1.0
- See Also:
getRowHeaderNodeFactory()
,setRowHeaderNodeFactory(Callback)
-
-
Constructor Details
-
GanttChart
public GanttChart()Constructs a new Gantt chart control.- Since:
- 1.0
-
GanttChart
Constructs a new Gantt Chart control.- Parameters:
root
- the root row of the Gantt chart- Since:
- 1.0
-
-
Method Details
-
createDefaultSkin
- Overrides:
createDefaultSkin
in classControl
-
displayModeProperty
A property used to specify the mode in which the Gantt chart will layout its primary views, the table and the graphics. Using this property the application can quickly switch between a standard, table-only, or graphics-only display.- Since:
- 1.0
- See Also:
getDisplayMode()
,setDisplayMode(GanttChart.DisplayMode)
-
setDisplayMode
Sets the value of thedisplayModeProperty()
.- Parameters:
mode
- the new display mode- Since:
- 1.0
-
getDisplayMode
Returns the value ofdisplayModeProperty()
.- Returns:
- the display mode (standard, table only, graphics only)
- Since:
- 1.0
-
createTreeTable
Creates the tree table view instance. Applications can override this method to return a customized table.- Returns:
- a tree table view instance
- Since:
- 1.0
-
tableMenuButtonVisibleProperty
This controls whether a menu button is available when the user clicks in a designated space within the TreeTableView, within which is a check menu item for each column in this table. This menu allows for the user to show and hide all TreeTableColumns easily.- Since:
- 1.0
- See Also:
isTableMenuButtonVisible()
,setTableMenuButtonVisible(boolean)
-
setTableMenuButtonVisible
public final void setTableMenuButtonVisible(boolean value)Sets the value oftableMenuButtonVisibleProperty()
.- Parameters:
value
- if true the menu button will be shown to the user- Since:
- 1.0
-
isTableMenuButtonVisible
public final boolean isTableMenuButtonVisible()Returns the value oftableMenuButtonVisibleProperty()
.- Returns:
- true if the table menu button is visible
-
rootProperty
Returns the root row property. The root row will become the root node of the Gantt chart's tree table control on the left-hand side (wrapped inside an instance of typeGanttChartTreeItem
). Other rows can be added by adding them to the root row or one of its children.- Since:
- 1.0
- See Also:
getRoot()
,setRoot(R)
-
setRoot
Sets a new root on the Gantt chart, which will cause the framework to set a new root of typeGanttChartTreeItem
on the underlyingTreeTableView
.- Parameters:
root
- the new root of the model- See Also:
rootProperty()
-
getRoot
Returns the root row of the Gantt chart.- Returns:
- the root row
- Since:
- 1.0
-
getRowHeaderColumn
Returns the row header control used as the first column of the tree table view. The row header displays line numbers, or level numbers, or any arbitrary graphics node.- Returns:
- the row header (column)
-
createRowHeaderColumn
Creates the row header column used by the Gantt chart. Applications can override this method to return a customized row header.- Returns:
- the row header column
- Since:
- 1.0
-
getTreeTableScrollBar
Returns the scrollbar that is being used for horizontal scrolling operations of the tree table view.- Returns:
- the horizontal tree table view scrollbar
- Since:
- 1.0
-
getTreeTable
Returns theTreeTableView
instance that is shown on the left-hand side of the Gantt chart.- Returns:
- the tree table view
- Since:
- 1.0
- See Also:
createTreeTable()
-
getTreeTableMasterDetailPane
public org.controlsfx.control.MasterDetailPane getTreeTableMasterDetailPane()Returns the primaryMasterDetailPane
instance that is being used to display theTreeTableView
and theListViewGraphics
.- Returns:
- the primary master detail pane
- Since:
- 1.6
-
showTreeTableProperty
A property used to control whether the tree table view will be shown or not. This node gets shown on the left-hand side of the Gantt chart and dispays a hierarchy (e.g. a resource hierarchy). The tree table view is the detail node of the primary master detail pane (seegetTreeTableMasterDetailPane()
).- Since:
- 1.0
- See Also:
isShowTreeTable()
,setShowTreeTable(boolean)
-
isShowTreeTable
public final boolean isShowTreeTable()Returns the value ofshowTreeTableProperty()
.- Returns:
- true if the tree table should be shown
- Since:
- 1.0
-
setShowTreeTable
public final void setShowTreeTable(boolean show)Sets the value ofshowTreeTableProperty()
.- Parameters:
show
- if true the tree table becomes visible- Since:
- 1.0
-
rowHeaderTypeProperty
A property used to store the currently used type of row headers (row number, level number, custom graphics).- Since:
- 1.0
- See Also:
getRowHeaderType()
,setRowHeaderType(GanttChart.RowHeaderType)
-
setRowHeaderType
Sets the value ofrowHeaderTypeProperty()
.- Parameters:
type
- the row header type (row number, level number, graphics)- Since:
- 1.0
-
getRowHeaderType
Returns the value ofrowHeaderTypeProperty()
.- Returns:
- the currently used row header type (line number, level number, custom graphics)
- Since:
- 1.0
-
rowHeaderNodeFactoryProperty
A property used to store a callback for creating a node that will be placed to the left of each row in the tree table view. This factory will only be invoked if the row header type is set toGanttChart.RowHeaderType.GRAPHIC_NODE
.- Since:
- 1.0
- See Also:
getRowHeaderNodeFactory()
,setRowHeaderNodeFactory(Callback)
-
setRowHeaderNodeFactory
Sets the value ofrowHeaderNodeFactoryProperty()
.- Parameters:
factory
- the factory used for creating the row header nodes- Since:
- 1.0
-
getRowHeaderNodeFactory
Returns the value ofrowHeaderNodeFactoryProperty()
.- Returns:
- the row header nodes factory
-
expandRows
public final void expandRows()Expands all rows inside the Gantt chart. This method will use recursion to find all rows that are parents and to expand them.- Since:
- 1.3
- See Also:
Row.setExpanded(boolean)
,expandRowsByOneLevel()
,collapseRows()
,collapseRowsByOneLevel()
-
expandRowsByOneLevel
public final void expandRowsByOneLevel()Expands the next level of rows inside the Gantt chart. This method will use recursion to find the first rows that are parents and currently not expanded. It will then expand it to make its children visible. Successive calls to this method will eventually show all rows.- Since:
- 1.3
- See Also:
Row.setExpanded(boolean)
,expandRows()
,collapseRows()
,collapseRowsByOneLevel()
-
collapseRows
public final void collapseRows()Collapses all rows inside the Gantt chart. This method will use recursion to find all rows that are parents and to collapse them.- Since:
- 1.3
- See Also:
Row.setExpanded(boolean)
,collapseRowsByOneLevel()
,expandRows()
,expandRowsByOneLevel()
-
collapseRowsByOneLevel
public final void collapseRowsByOneLevel()Collapses the hightest level of rows inside the Gantt chart that is currently being used. Successive calls to this method will eventually close the entire tree.- Since:
- 1.3
- See Also:
Row.setExpanded(boolean)
,expandRows()
,collapseRows()
,collapseRowsByOneLevel()
-
resizeColumns
public final void resizeColumns()This method will resize all columns in the tree table view to ensure that the content of all cells will be completely visible. Note: this is a very expensive operation and should only be used when the number of rows is small.- Since:
- 1.3
- See Also:
resizeColumn(TreeTableColumn, int)
-
resizeColumns
public final void resizeColumns(int maxRows)This method will resize all columns in the tree table view to ensure that the content of all cells will be completely visible. Note: this is a very expensive operation and should only be used with a small number of rows.- Parameters:
maxRows
- the maximum number of rows that will be considered for the width calculations- Since:
- 1.3
- See Also:
resizeColumn(TreeTableColumn, int)
-
resizeColumn
This method will resize the given column in the tree table view to ensure that the content of the column cells will be completely visible. Note: this is a very expensive operation and should only be used when the number of rows is small.- Parameters:
column
- the column that will be resized- Since:
- 1.3
- See Also:
resizeColumn(TreeTableColumn, int)
-
resizeColumn
This method will resize the given column in the tree table view to ensure that the content of the column cells will be completely visible. Note: this is a very expensive operation and should only be used when the number of rows is small.- Parameters:
tc
- the column that will be resizedmaxRows
- the maximum number of rows that will be evaluated for the width calculation- Since:
- 1.3
- See Also:
resizeColumn(TreeTableColumn, int)
-